26 Mar 2015
I love programming, I really do. I like making stuff work, I like when I finally wrap my head around a concept, I really like rubber-ducking with people. Since I love it so much, I can get pretty excited when someone tells me they’re learning to program. And I get even more excited when they ask me for advice. Vainly, it makes me feel good that someone thinks I know enough that they might want to take my advice.
Often people ask “What do you wish you had known when you were #{insert stage/job/etc here}?” So this is “Letters to a Young Developer”. The series of blog posts and advice I wish I’d come into when I was first #{insert stage/job/etc here}.
What Should I Learn Next?
“What should I learn next?” This was the question I asked once I’d finished a few of the recommended tutorials for new Ruby/Rails developers. At that time I was thinking I needed to practice certain techniques, learn how to implement certain features. For a long time when people asked me what they should learn next I would rattle off a laundry list of my favorite tutorials and guides. I assured them (as I had been assured myself not so many months before) that picking up another programming language was easy after the first one or two.
What I didn’t think about though, was why it was easier to pick up another language after you had already learned one. I had the benefit of having a little bit of experience on my side, so I was familiar with the concept of debugging. What I didn’t realize that what I was missing was understanding how to effectively debug in Ruby.
As I get more opportunities to work with other beginners, the difficulties of really learning and understanding a first language become much clearer to me. Even across different learning types, it seems like beginners’ struggles circle around a few themes:
- recognizing patterns
- understanding syntax
- figuring out what went wrong
The first two, I think can be learned through writing code and reading. The last one is a little trickier. Sure, if you know a lot about design you can probably reason your way through what you think went wrong. But even with tests in place, sometimes your best guess is just that, a guess.
What I Wish I Knew
What I wanted to learn when I was asking “What should I learn next?”, was really “How can I learn what’s really going on?”. My problem was I could implement the same things I had implemented before but I was nervous about implementing new things as I wasn’t sure how to do them without a guide. Because at the end of the day, when you understand the language you can really learn how to implement almost any feature.
Think about when you work with someone (or when you worked on things) and something goes wrong they(or you) say “That keeps happening & I don’t know why”. They’ve tried this several times before and gotten the same error but the error doesn’t mean anything to them because they don’t know how to read it. This was me when I was first starting to write applications outside of the safety and guidance of tutorials. I knew something was wrong but I didn’t know how to troubleshoot it.
Learning to Troubleshoot
The advice I’d give young Kylie (just a year & a half ago) would be learn how to troubleshoot. The very first thing I’d recommend to learn how to do this would be watching Blithe Rocher’s Scientific Method of Troubleshooting. Developers seem to value methodical approaches to problem solving, so this is the perfect marriage of development and learning. For other new Ruby developers I’d recommend Jonathan Wallace’s Effective Debugging in Ruby and this presentation by Melissa Holmes on Pry for Ruby. Learning how to debug effectively can make the difference between feeling like you’re floundering and being able to move on quickly from mistakes. I know for me at least, being able to debug on my own has helped a ton. One, because you’re able to power through problems and figure out what’s really going down. Two, because of the great confidence boost that comes from finding a problem in code and fixing it yourself.
Letters to a Young Developer
This was just part one of a series called “Letters to a Young Developer” where I’ll share the great advice I got, or the advice I wish I’d gotten when I first started learning Ruby. Maybe these posts will be helpful to someone, but if not I’ll at least have a good log of my experiences.
16 Feb 2015
I’m trying to keep track of the tools I use most and also remember how to use them. Since I have a terrible memory I’m doing the thing where you write you blog posts as reminders for yourself so here’s the first one:
Hub
What is Hub? Hub is a command line tool that wraps git
in order to extend it with extra features and commands that make working with GitHub easier. (Ed note: A+ copy-pasting Kylie!). The hub project recommends you alias git
to hub
but I prefer not to do that so I can remember which are hub
commands and which are git
commands.
I mostly use hub for creating pull requests from the command line and for creating GitHub remotes. I still use a few gui-based tools for development(namely my editor), but I’m getting comfortable with more “keyboard-only” tools and feel I’m able to move faster when I use them.
Hub Pull-Request
Hub’s pull-request
is the command I use most. hub pull-request
allows you to create a pull request between your current branch and any other branch on your project. This is particularly handy for me because my workflow for work involves feature branches that are merged into a staging
branch where features are accepted or rejected before being merged into a master
branch that goes into production.
Despite the fact that I use this command near daily, I almost always forget that I need to push my branch to the GitHub remote to create a pull request. Just like using the GitHub web gui you can’t create a pull request for a remote that doesn’t exist. Here’s the workflow I use when I write features:
- Check out a feature branch
$ git co -b kyfast/excellent-feature
-
Write & commit code
-
Push feature branch to remote. I should also note I have git configured to automatically set up remotes when I check out a new branch on existing GitHub projects.(Ed note: this is the part you always forget)
$ git push
- Open Pull-Request from the command line. There are a few options you can pass in her but I usually just use -b to indicate which branch I want to open my pull request against:
$ hub pull-request -b staging
That command opens up your git config editor so you can write a pull request title:
0
1 # Requesting a pull to kyfast:staging from kyfast:excellent-feature
2 #
3 # Write a message for this pull request. The first block
4 # of text is the title and the rest is description.
all done! Hub will spit out the url of the pull request in your terminal so you can check it out online.
Hub Create
My second most used hub
command is create
. hub create
creates a github remote for you on your default account in any git repository. Here’s the basic workflow from within your project’s directory:
- Initialize git repo(let me know if I’m going too fast):
$ git init
- Create a remote with hub:
$ hub create
that’s it. That’s the whole thing. Hub will spit out a confirmation that your remote has been created:
Updating origin
created repository: KyFaSt/excellent_project
Hubbub About Hub
If you are already using git-cli to interact with GitHub and enjoy it, I recommend you install and try out hub. It’s easy to use and saves extra clicks that you should be using on cookies instead.
16 Nov 2014
In September, I attended the Strange Loop Conference in St. Louis by way of their diversity scholarship. I’ll admit I was a little nervous about attending as a Ruby developer, as I’d overheard the conference’s unoffical byline: “an annual event to destroy object oriented progamming”. I got to experience a lot of awesome things and meet really cool people but in the interest of brevity I’ve only shared a few of my favorite experiences here.
Pre-Conference: Powered by JavaScript
There were a lot of awesome sessions scheduled for the day before the conference began, including a full day JavaScript hosted by Manning Publications, which I attended. I’m a web developer but JavaScript is not my strong point so I got to see a lot of really interesting & thought provoking talks. They even gave everyone who pre-registered a copy of their Single Page Web Applications book I think one of my favorites was Sarah Groff-Palermo’s talk on Art.JS. I really enjoy seeing people do creative things with what is ordinarily considered a creative toolset, like programming.
I also caught a talk on React.JS that I enjoyed. I have dabbled with some JavaScript frameworks but React.JS is supposed to be a bit more functional so I think after the course I’m currently taking is finished, I’ll probably start learning about React.
Favorite Sessions
All of the talks I saw were really awesome, but I did have 2 favorites by far. I think I liked them both because they made functional programming feel much more accessible to me.
Felienne Hermans on Excel for Programmers
Felienne Hermans’ talk on Excel for programmers was one of my favorite talks. Initially, I didn’t want to attend because I got my start in programming writing VBA in Excel & I didn’t really want to dredge up those memories. Once I read the talk description, I knew I couldn’t miss it. Felienne proved that Excel was a programming language and that it was also a very good functional programming language.
What was most amazing to me was seeing the test suites she was working on for Excel, people might joke about being “Excel Jockeys” but in all truth, these spreadsheets can become complex financial decision making systems that need to have verifiable data. Which is, in my opinion, the perfect scenario to implement a test suite. Afterwards, I spoke with her & joked that if I had found out about her research before I found out about Ruby, I’d probably be a grad student helping write a test framework for excel.
Julia Evans’ “You Can Be a Kernel Hacker!”
This talk was pure fun from start to finish. I was concerned that the “you” in the talk title was a functional programmer, but I figured I’d survived (& understood some) the talks I’d heard up until then so I might be alright. Julia did a great job of breaking down the scary parts of kernel hacking & making the audience feel comfortable.
My favorite part of this talk was actually Julia’s response to one of the questions. When someone asked how she kept from wrecking her system while working on this, she said “I didn’t” & everyone laughed. It was really cool that she was comfortable admitting that in front of a huge crowd of programmers and all of them thought it was funny that she didn’t provide a detailed technical answer.
Lambda Ladies
After the first full day of the conference, and before the pre-conference party at St. Louis’ City Museum there was a party hosted by Lambda Ladies. It was really nice to meet women who write functional languages on the regular and I didn’t feel as nervous about going to the pre-conference after making a few more new friends.
A Strange Loop Indeed
After the conference, I knew I wanted to learn more about functional programming but I wasn’t sure where to get started. A friend suggested we check out Deltfx’ Intro to Functional Programming edx course which is taught using the Haskell language, and once again I’ve been pleasantly surprised by Functional Programming. It’s only a 6 week course, but I think that if I hadn’t attended Strange Loop this year there is probably no way I’d have registered for the course.
Before Strange Loop, I was afraid that functional programming wasn’t for me and I wouldn’t fit in because I didn’t know very much. But I found that while I was there, that by being a developer who gets excited about learning and stretching my brain in new ways that Strange Loop and Functional Programming are for me (& probably for you too!)
15 Aug 2014
You might have a lot of reasons to participate in a hackathon but one of my goals is to learn something new. For me at least, I hope that something new will be how to implement a feature I haven’t before or get more comfortable with an unfamiliar language. To make it easier to learn something new, I have picked up a few things that help me reduce distractions and focus on writing code.
Stop
Before I get ahead of myself thinking about the awesome feature-packed app I want to build, I try to take a minute to make sure I have all the tools I need. For me, that includes making sure I’ll be able to make something that not only works well internally but also appeals to users.
I like to try to find someone with front end development or design experience to work with, then my app looks great and I’ve met someone new. A site with appealing design confers professionalism and an essence of completeness to judges.
If you’re unable to find a designer to work with, and you promise you really tried (sometimes I get too shy to ask anyone, so that doesn’t count) you can always use some prepackaged styling. The biggest mistake I’ve made before in this area is thinking it’s easy and waiting until the last minute to “slap bootstrap on there.” I try now to include styling as one of the first few steps after rails new
. Twitter Bootstrap is very popular but I also like Zurb Foundation.
Collaborate
Working with other developers, designers & possibly non-technical team members can be challenging especially if you feel like you don’t speak the same language.
Encourage teammates to focus on single responsibility additions to your code base by using git branches for features. This makes it easier to merge in individual features, and helps to keep each contributor laser focused on one task at a time. Additionally if someone needs to trade off of a feature, someone else can pull down that feature branch to get just the code they need.
Keeping track of all of those feature branches can be time consuming, and possibly complicated for less technical teammates. A friend introduced me to a chat room-like software called Gitter, which integrates with Github, Bitbucket, Trello, Travis & several more code/project management tools. It has a chatroom & shows a feed on the right of recents commits, pushes & updates. I think it is a great way to integrate non-technical team members into the development process & can reduce possible “us vs. them” mentality.
Listen
At the end of a hackathon, each team is usually asked to create a 5-10 minute demo of their work. Some teams might be scrambling to implement features or others might be there physically but maybe not so there mentally. I try to think of things that will make it easier for an audience to watch the presentation, or if the final product allows it, try out the app themselves.
The first thing is pre-populating the database, either by writing some custom rake tasks or using the ruby gem faker. Filling the app with some information helps the audience visualize how it will look in production as well as really making any of features based on user-input or data analysis shine.
Another thing I like to keep in mind is preventing the user from seeing the dreaded Heroku “We’re sorry, something went wrong” page. This can happen for any number of reasons but recently I’ve experienced it by letting a user pass a nil value to a required field. To prevent this in the future, I like to user either HTML5 validations or a rails formbuilder like Formtastic or Simple Form which select the user input field type based on the database column-type. The form builder options can really come in handy when you don’t have a dedicated designer on your team.
A Brand New Invention
A lot of what I’ve collected above is pretty rails-focused, because that’s primarily what I’ve worked with during hackathons (excluding a misguided but semi-successful attempt at JavaScript). Hopefully I’ll be able to find some tools to make features my main focus in other languages and frameworks soon. For now though, I’m pretty excited about the idea of doing some purely ruby based hacking. If you are too, you should join me at Big Nerd Ranch’s Ruby Data Structure Hack Night on Monday August 25th 2014!
08 Aug 2014
Dang
You might be thinking “dang, this is a pretty good looking site.”
You would be correct in thinking that, this site looks pretty good. Thanks to a sweet new theme and a few simple tools, refreshing my site was easy and painless.
This site is built with jekyll so the first thing I did was select a proper theme. Previously I’d cobbled together the css for my site on my own and without any design experience, it looked messy and busy. I chose the hyde theme by @mdo because of how simple it looks. But because it actually has a fairly simple structure it provides the flexibility to customize later.
I chose to continue hosting through GitHub Pages because pushing changes to my live site is just as simple as git push
. This is a fairly simple setup (not to mention easy to maintain) but without a domain name, the site still sits as a subdomain of github. There’s certainly nothing wrong with that but I think I’d like something a bit fancier.
That’s where the fun part comes in. I’d actually never purchased a domain before or put a site on the web that wasn’t a rails or javascript app so this is foreign territory. Fortunately with GitHub Pages & the newly introduced Google Domains* setting up a custom domain is virtually effortless.
The Hard Part
Just kidding, this was a walk in the park. First things first, I purchased the kyfast.net domain name from Google Domains for a reasonable price of $12/yr.
In the root folder of my project I created a file called CNAME
that only contains the domain name I want my site to be hosted at:
I pushed this up to the master branch on github & then checked on the repo’s settings that changes would be pushed to my domain:
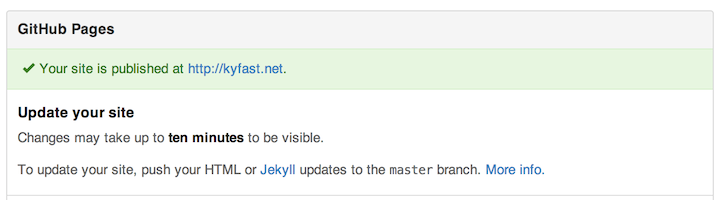
That wasn’t too bad, just one step remained: pointing the the domain to the web server where my site was hosted. This part was a little trickier, I knew I needed to set up a custom resource record but I wasn’t certain which type to use.
After some searching through GitHub Pages’ help resources I found this page. Here I determined I could set up my custom resource route as type ‘A’. After navigating to the ‘advanced’ section of this domain’s management page I input the IPs provided from the GitHub Pages help:
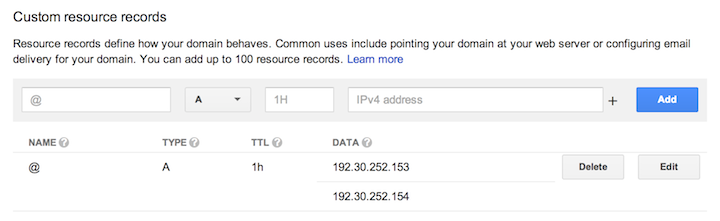
I ran dig kyfast.net
from my command line & returned the two IPs I set in the resource record:
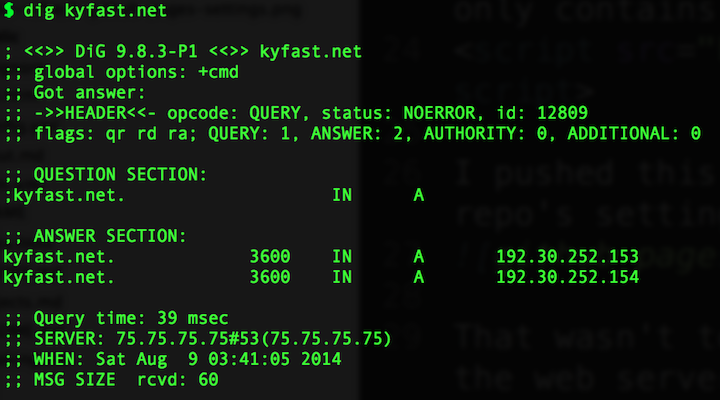
Alternatively, you can always just hit your site & make sure it’s serving the content you expect.
Retrospective
Updating and maintaining my personal site has been a frustrating experience until now. I thought because I’m a developer I should be able to figure out how to do the design piece of a website, but I’m not at that point in design yet. By really simplifying the theme and being realistic about what I need my site to do, I’m able to screen out distractions (like my old garish color schemes) & focus on the content that matters.
*Google Domains is still in invite-only beta mode as of 08-08-2014